"Mastering Python's Generators: A Comprehensive Guide"
Blog post description.
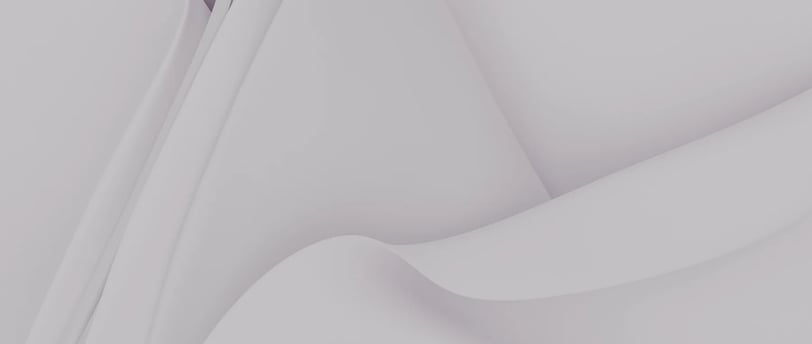
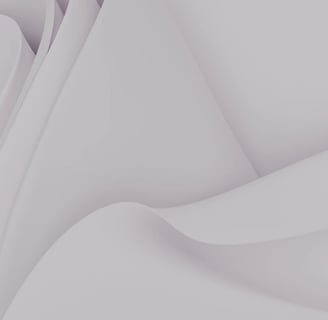
One essential and potent component of Python programming are generators. They make it possible to create iterators that generate values dynamically, facilitating lazy evaluation and effective memory usage. We will go further into the realm of Python generators in this extensive guide, covering its syntax, applications, advantages, and sophisticated methods.
Introduction to Generators
Fundamentally, generators are unique functions that generate a succession of values by using the `yield` keyword. In contrast to standard functions, which terminate and return a value to the caller via `return}, generators can yield many values one at a time, halting and continuing execution as necessary.
Let's start by examining the basic syntax of generators:
python
def my_generator():
yield 1
yield 2
yield 3
In this example, `my_generator` is a generator function that yields the values 1, 2, and 3 in sequence. When called, it returns a generator object that can be iterated over to retrieve each value.
Understanding Generator Execution
A generator function does not execute its body when it is called; instead, it returns a generator object. When the `next()` method is called on the generator object, or when the generator function is iterated over using a loop, the generator function is executed.
python
gen = my_generator()
print(next(gen)) # Output: 1
print(next(gen)) # Output: 2
print(next(gen)) # Output: 3
Each call to `next()` resumes the execution of the generator function until the next `yield` statement is encountered, at which point the value is yielded back to the caller.
Benefits of Generators
1. Memory Efficiency: Values are generated by generators in a lazy manner, or solely in response to requests. This makes it possible to use memory effectively, especially when working with big datasets or endless sequences.
2. Lazy Evaluation: Because values are produced dynamically as needed, processing of data streams or infinite sequences is possible without requiring advance calculation.
3. Composability: Complex data processing pipelines can be built by combining generators with additional iterators and generator expressions. They may therefore be applied to a broad variety of use situations and are extremely versatile.
Generator Expressions
Generator expressions, which offer a condensed method of creating generators without the requirement for a separate function specification, are supported by Python in addition to generator functions.
python
gen_expr = (x 2 for x in range(5))
for num in gen_expr:
print(num)
This example creates a generator that yields the squares of numbers from 0 to 4. Generator expressions are especially useful for simple transformations or filtering operations on iterable data.
Advanced Generator Techniques
1. Sending Values to Generators: Generators can use the `send()` method to accept values from the caller in addition to returning data. More sophisticated behavior is made possible by the twoway communication this permits between the generator and its caller.
2. Exception Handling: By incorporating a `tryexcept} block within the generator function, generators may handle exceptions that are raised during iteration. This enables the generator to gracefully handle errors and recover from them.
3. Nested Generators: Because generators are nestable, multistep processing pipelines and hierarchical data structures can be constructed.
RealWorld Examples
Let's explore a few real world examples to demonstrate the practical applications of generators in Python programming.
1. Reading Large Files: Large files can be read line by line using generators, which handle each line separately without requiring the full file to be loaded into memory.
2. Infinite Sequences: Generators are perfect for producing prime numbers or other infinite sequences without requiring you to compute all of the values in advance, like the Fibonacci sequence.
3. Asynchronous Programming: Asynchronous programming patterns can be implemented with generators, which makes it possible to handle concurrent tasks effectively without the hassles of callbacks or threads.
Advanced Generator Techniques
Python generators provide a wealth of sophisticated methods that can help you become a better coder. Using generator expressions, which are condensed and memory efficient substitutes for list comprehensions, is one such method. With the use of these expressions, generators can be created directly without the requirement for a distinct function declaration. As an illustration:
python
# Generator expression to generate squares of numbers from 0 to 9
squares = (x2 for x in range(10))
Generator expressions are particularly useful when you need to iterate over a sequence once and don't need to store the entire result in memory. They can be combined with other iterable functions like `filter()` and `map()` to create powerful data processing pipelines.
Another advanced feature of generators is their ability to receive values from the caller using the `send()` method. This twoway communication mechanism allows for more dynamic and interactive generator behavior. For instance, you can implement a generator that generates Fibonacci numbers based on input from the caller:
python
def fibonacci():
a, b = 0, 1
while True:
value = yield a
if value is not None:
a, b = value, a + b
else:
a, b = b, a + b
# Using the Fibonacci generator
fib = fibonacci()
print(next(fib)) # Output: 0
print(fib.send(10)) # Output: 1
print(next(fib)) # Output: 1
print(next(fib)) # Output: 2
In this example, the `fibonacci()` generator produces Fibonacci numbers indefinitely. The `send()` method allows the caller to send a value to the generator, which then uses it to modify its internal state accordingly.
Exception handling is another essential aspect of generator programming. Generators can catch exceptions raised during iteration and handle them gracefully using a `tryexcept` block. This allows for robust error handling within the generator itself, enhancing code reliability and maintainability. For example:
python
def process_data(data):
for item in data:
try:
# Process the item
result = do_something_with(item)
yield result
except Exception as e:
# Handle the exception gracefully
print(f"Error processing item: {item}. Reason: {e}")
# Using the generator with error handling
data = [1, 2, 'a', 4, 5]
for result in process_data(data):
print(result)
In this snippet, the `process_data()` generator iterates over a sequence of items and processes each item. If an exception occurs during processing, it is caught and handled within the generator, ensuring that the iteration continues without interruption.
Conclusion
Python's generators are a strong and adaptable feature that allow for effective iteration and lazy data evaluation. Your code will be more expressive, memory efficient, and reliable if you can write sophisticated generating techniques and master their utilization. Generators offer a versatile and sophisticated resolution to several programming problems, regardless of the complexity of the method, the size of the dataset, or the infinite sequences involved. Try out several generators in your Python projects to see how they might improve your coding skills and output. Have fun with coding!