Exploring Python's Concurrency and Parallelism: Multi-Threading, Multi-Processing, and AsyncIO
Blog post description.
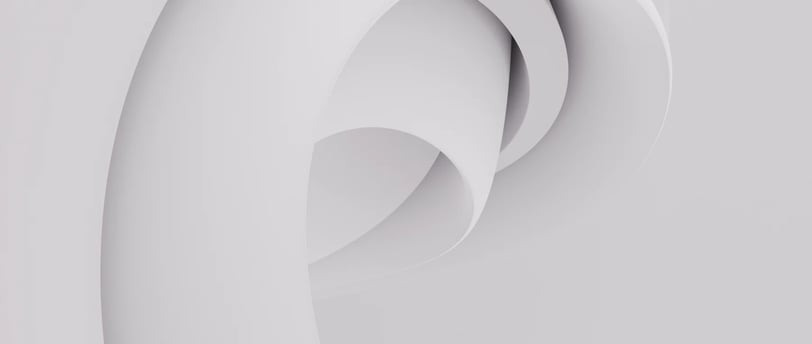
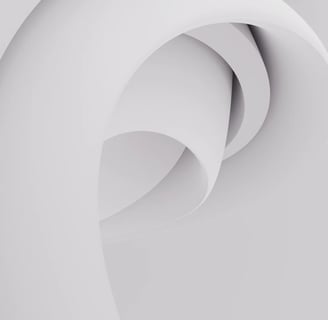
Modern software development relies heavily on the principles of concurrency and parallelism, which allow programs to do numerous tasks at once and make optimum use of computer resources. To achieve concurrency and parallelism in Python, developers can utilize multiple methods and modules, such as AsyncIO, multi-processing, and multi-threading. We'll examine each of these strategies in detail in this blog article, looking at their advantages, disadvantages, and applications in Python development.
Understanding Concurrency and Parallelism
The ability of a program to carry out numerous activities at once, or concurrency, enables it to advance on multiple tasks at once. In contrast, parallelism refers to the use of several CPU cores or processors to carry out multiple tasks at the same time. Although these ideas are similar, they are not the same in terms of how resources are used and activities are carried out. Python concurrency and parallelism are powerful tools that developers may use to enhance the responsiveness and performance of their programs, particularly when working on CPU- or I/O-bound tasks.
Multi-Threading in Python
A concurrency mechanism known as multi-threading allows for the sharing of memory between several threads of execution operating within a single process. With the help of Python's threading module, developers may easily establish and manage threads and carry out concurrent activities without interfering with the main thread of execution. Although multi-threading can enhance the speed of I/O-bound operations, its application to CPU-bound tasks is restricted because of Python's Global Interpreter Lock (GIL), which forbids the simultaneous execution of Python bytecode by multiple threads.
Multi-Processing in Python
A parallelism model known as multi-processing allows for the simultaneous operation of several processes, each with its own memory space. With the help of Python's multiprocessing module, developers may establish and oversee numerous processes, utilizing multiple CPU cores to carry out tasks concurrently. Python code that uses multi-processing, as opposed to multi-threading, can avoid the GIL and is therefore appropriate for CPU-bound activities involving large amounts of computation. Developers can improve efficiency and scalability by utilizing the full computing capability of contemporary multi-core processors by dividing work over numerous processes.
AsyncIO in Python
With the use of coroutines and event loops, AsyncIO, a concurrency framework first introduced in Python 3.4, allows for asynchronous I/O operations. In contrast to parallelism-based multi-threading and multi-processing, AsyncIO accomplishes concurrency by enabling tasks to willingly relinquish control of execution while awaiting the completion of I/O operations. Web servers and network clients are two examples of network-bound programs that benefit greatly from this non-blocking I/O paradigm since they demand high concurrency and scalability. Developers may create asynchronous code that is very effective and scalable using AsyncIO, all without the hassle of conventional multi-threading or multi-processing models.
Choosing the Right Approach
To sum up, Python provides programmers with a variety of parallelism and concurrency choices, each with unique advantages and disadvantages. Developers should take into account various aspects, including the tasks' nature, the desired amount of concurrency, and the hardware's performance characteristics, when selecting the best method for a given use case. Python developers may efficiently use concurrency and parallelism to create high-performance, scalable, and responsive applications by knowing the concepts and capabilities of multi-threading, multi-processing, and AsyncIO.
Concurrency Control and Shared Resources
Managing shared resources and preventing race situations present a difficulty for Python developers implementing concurrency. When many threads attempt to access shared data at the same time in multi-threaded programs, unpredictable behavior and possible data corruption may result. Python has tools to synchronize access to shared resources and avoid race situations, including locks, semaphores, and queues. In multi-threaded and multi-processed applications, developers can guarantee the consistency and integrity of shared data by skillfully utilizing these concurrency control primitives.
Scalability and Performance Considerations
Scalability and performance implications are important factors to take into account when creating concurrent Python applications. Concurrency can increase an application's responsiveness and performance, but it can also add overhead and complexity. For their concurrent algorithms to minimize contention, maximize parallelism, and prevent bottlenecks, developers need carefully design and optimize them. Better scalability and performance in multi-core or distributed systems can be achieved by distributing work evenly among threads or processes through techniques like load balancing, workload segmentation, and asynchronous processing.
Error Handling and Debugging
Because concurrency-related issues are non-deterministic, debugging concurrent Python programs can be difficult. In multi-threaded or multi-processed programs, frequent problems that can occur include race situations, deadlocks, and thread hunger. Python has debugginggers, profiling tools, and logging to assist developers in identifying and resolving concurrency-related issues. Furthermore, using best practices like comprehensive testing, code reviews, and defensive programming can aid in detecting and averting concurrency problems early in the development process.
Asynchronous Programming with AsyncIO
Python 3.4 introduced AsyncIO, an asynchronous programming framework that makes it easier to create scalable and highly concurrent systems. AsyncIO achieves parallelism without the burden of thread or process management by utilizing coroutines and event loops, in contrast to conventional multi-threading or multi-processing approaches. Web servers, chat programs, and real-time data processing systems are examples of I/O-bound applications that benefit greatly from asyncIO. Developers can design effective, non-blocking programs that can manage thousands of concurrent connections with little resource overhead by utilizing AsyncIO.
Hybrid Approaches and Best Practices
A hybrid strategy that combines many concurrency approaches might occasionally provide the optimal combination of simplicity, scalability, and performance. For instance, developers can utilize AsyncIO to handle I/O-bound operations asynchronously and multi-threading or multi-processing to parallelize CPU-bound processes. Based on the needs of their applications, developers can carefully choose and combine concurrency approaches to maximize performance and resource consumption while preserving readability and simplicity of code. Furthermore, implementing best practices like error handling, testing, and appropriate resource management can help guarantee the dependability and maintainability of concurrent Python applications.
Concurrency Patterns and Design Considerations
Developers should become familiar with popular concurrency patterns and recommended practices in addition to comprehending concurrency primitives and methods. Producer-consumer, thread pool, and worker pool are a few examples of patterns that can be used to efficiently manage resources and organize concurrent applications. Concurrent Python application design and implementation also heavily depend on design factors including task granularity, data sharing and synchronization, and error handling techniques. Developers can create concurrent solutions that match application needs with robustness and maintainability by adhering to established patterns and taking design principles into consideration.
Real-World Applications of Concurrency in Python
Many real-world applications, such as web servers, network services, scientific computing, and financial analysis, depend heavily on concurrency. Concurrency is used, for instance, by web servers to manage several client requests at once, increasing scalability and responsiveness. Similar to this, concurrency is used by scientific computing applications to parallelize computations and speed up data processing. Concurrency is used in the financial industry to handle massive amounts of market data in real-time and effectively carry out algorithmic trading techniques. Developers can create responsive, scalable, high-performance programs that satisfy the demands of contemporary computing environments by utilizing concurrency.
Concurrency in Python Ecosystem
Concurrency is used by the many modules and frameworks in the Python ecosystem to effectively tackle a wide range of issues. For instance, concurrency is used by frameworks like Django and Flask to manage several HTTP requests at once, offering platforms for developing high-performance web applications. Concurrency is used by libraries like NumPy and SciPy to parallelize mathematical operations and speed up scientific computing workloads. Furthermore, developers can create asynchronous web servers and APIs that can manage thousands of concurrent connections with no resource overhead by using asynchronous libraries like Quart and aio http. Developers may efficiently utilize concurrency in their Python projects by utilizing these tools and modules.
Challenges and Trade-offs
Although concurrency has numerous advantages, there are trade-offs and difficulties that developers need to be aware of. In concurrent applications, for instance, controlling shared resources and preventing race circumstances can be difficult and error-prone. Concurrency can also result in overhead from synchronization overhead and context switching, which makes code more difficult. Furthermore, because of their non-deterministic behavior, concurrent applications can be difficult to debug and test. Developers can create reliable and effective concurrent solutions that satisfy application requirements by having a thorough understanding of the trade-offs and difficulties related to concurrency.
Future Trends and Advances in Concurrency
With continuous research and development aimed at enhancing scalability, performance, and usability, Python concurrency has a bright future. New technologies like asyncio, async/await syntax, and distributed computing frameworks like Ray and Dask are opening doors for concurrent applications that are more scalable and efficient. Furthermore, Python's parallelism and concurrency are being made possible by hardware innovations like GPUs and TPUs, which are specialized accelerators with multiple cores. Developers may continue to use concurrency to create scalable, responsive, and high-performance Python apps by keeping up with these trends and advancements.
Conclusion
In conclusion, investigating the concurrency and parallelism methods available in Python, such as multi-threading, multi-processing, and AsyncIO, uncovers an abundance of resources and approaches for creating scalable and effective programs. Because every strategy has advantages and disadvantages, developers must know when and how to use each one wisely.
Modern software development relies heavily on concurrency and parallelism, which allow programs to manage several activities at once and make effective use of computer resources. Concurrency is essential in a wide range of applications, from enhancing web server responsiveness to speeding up data processing in scientific computing to maximizing performance in financial analysis.
Python's extensive library and framework ecosystem, along with its support for concurrency primitives and patterns, enable developers to effectively handle challenging concurrency problems. Python provides a flexible toolkit for creating reliable and effective concurrent applications, from managing shared resources and preventing race situations to maximizing speed and scalability.
Future trends and advancements in concurrency promise even greater prospects for scalability, performance, and simplicity of use as developers continue to study and innovate in the field. Python's parallelism and concurrency are expanding due to new technologies like asyncio, distributed computing frameworks, and hardware innovations.
In today's fast-paced computing environment, developing high-performance, scalable, and responsive applications essentially requires an understanding of Python's concurrency and parallelism capabilities. Developers may generate significant impact in their projects and companies and uncover new avenues for innovation by mastering the concepts, patterns, and best practices of concurrency.