Mastering Python: Essential Tips and Tricks for Efficient Coding
Blog post description.
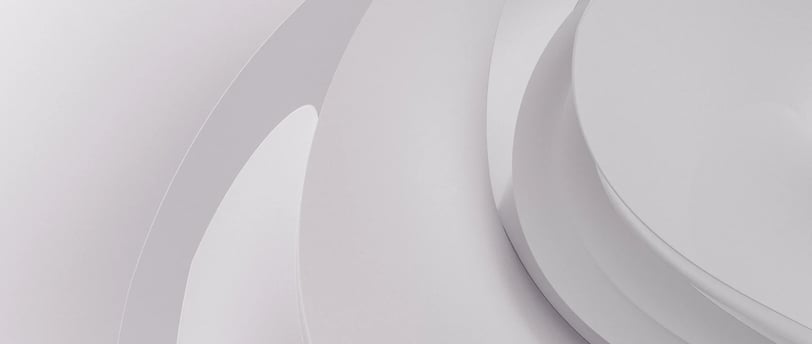
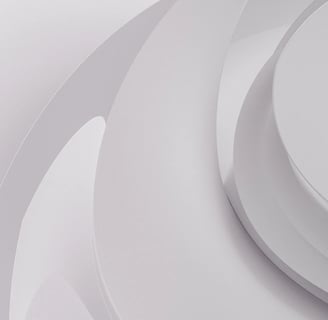
Introduction:
Python is one of the most widely used programming languages in the world; it is well-known for being straightforward, readable, and flexible. Understanding Python may greatly improve your productivity and efficiency, regardless of your experience level with coding. We'll cover all the important hints and techniques in this in-depth tutorial to help you write Python code that is clearer and more effective, enabling you to solve challenging issues with ease.
1. Embrace Pythonic Idioms:
Pythonic code follows the guidelines set out by the Python language, placing a strong emphasis on readability, elegance, and simplicity. Writing code that adheres to the standards and recommended practices of the Python community is a necessary part of embracing Pythonic idioms. This entails following PEP 8 style requirements, utilizing descriptive variable names, and avoiding creating new functions and modules in favor of using pre-built ones.
For instance, Python promotes the usage of list comprehensions and generator expressions in place of creating laborious loops to traverse over a list. These constructs improve the readability and efficiency of your code by helping you to express complex operations succinctly and effectively.
2. Leverage Built-in Functions and Libraries:
Python has an extensive built-in library with many modules and functions for a wide range of activities. Getting to know these built-in features will help you save time and effort when you're coding.
For example, the `collections` module provides specialized data structures that may be used to simplify typical programming chores, like `Counter`, `defaultdict`, and `deque`. In a similar vein, the `itertools` module offers functions for building iterators and combining them in potent ways to facilitate effective data manipulation and iteration.
To simplify typical programming chores, the `collections` module provides specialized data structures including `Counter`, `defaultdict`, and `deque`. Comparably, functions for building iterators and combining them in effective ways are provided by the `itertools` module, allowing for effective data manipulation and iteration.
3. Optimize Performance with Built-in Data Structures:
A wide variety of pre-built data structures are available in Python, each with unique use cases and performance attributes in mind. Composing code that is more scalable and efficient can be achieved by being aware of the advantages and disadvantages of various data structures.
For example, lists are often used and versatile for storing elements in homogenous sequences. Lists, however, might not perform as well when working with huge datasets or regularly changing items. Alternatives with quicker lookup and insertion speeds, like sets or dictionaries, might be more appropriate in some situations.
Similarly, performance can be greatly affected by selecting the right data format for your computational operations. For instance, constant-time lookup can be facilitated by storing key-value pairs in a dictionary, but linear time may be needed when looking through a list.
4. Embrace Functional Programming Paradigms:
Programming paradigms such as functional programming minimize mutable states and side effects by treating computing as the evaluation of mathematical functions. With capabilities like lambda functions, map, filter, and reduce, Python supports functional programming paradigms even though it is predominantly an imperative and object-oriented language.
You can create functions that are more reusable, expressive, and succinct by writing your code using functional programming principles. For instance, you may operate on collections with less boilerplate code by utilizing lambda functions and higher-order functions like `map` and `filter`.
Furthermore, you can perform slow evaluation and manage big datasets effectively thanks to Python's support for generators and generator expressions. You can write code that is more modular and composable, which makes code reuse and maintenance easier, by adopting functional programming principles.
5. Adopt Test-Driven Development (TDD) Practices:
Writing tests prior to writing code is emphasized in the software development methodology known as "test-driven development," or "TDD." You can make sure that your code satisfies the requirements and is strong and dependable throughout its lifecycle by adhering to TDD principles.
Comprehensive support for authoring and running tests is offered by Python testing frameworks such as doctest, pytest, and unittest. With the help of these frameworks, you can automatically run tests, specify test cases, and assert expected results to validate that your code is correct.
Writing modular, decoupled code with distinct interfaces is another benefit of using TDD techniques; this makes the code simpler to maintain and refactor. You may reduce the chance of regressions and improve the overall quality of your codebase by minimizing the number of problems you find and fixing early in the development process with tests.
Conclusion:
Learning Python is a journey that calls for inquiry, practice, and constant learning. You can increase your productivity and efficiency as a Python developer by implementing the crucial pointers and techniques provided in this tutorial. Write cleaner, more efficient code and confidently take on challenging challenges with these tactics, which can be applied to performance optimization, Pythonic idiom adoption, or functional programming paradigms. Recall that experimentation, iteration, and a dedication to lifelong learning are essential to become a proficient Python programmer. Have fun with coding!